Last Updated on May 19, 2025
For advanced IoT projects some times we need Integration of Microcontroller with modern communication platform. One such case is Interfacing ESP32 board with WhatsApp to send message or data from sensor. For real time notification, remote monitoring and IoT automation, this interface will help a lot. Let’s make ESP32 WhatsApp message Interface by simple and easy to follow steps.
ESP32 Microcontroller board is the best suitable device for IoT projects and IoT experiments. Because of its internal Wi-Fi and Bluetooth capability. Here we are going to use ESP32 microcontroller Wi-Fi feature to send WhatsApp messages by using third party API Service called CallMeBot which is free to use. If you want scalable and backend support you can use Twilio. ESP32 microcontroller connect to Wi-Fi network and make HTTP request to the API (CallMeBot) and then send a predefined message to a specific WhatsApp Number.
Circuit & Wiring
We are going to use ESP32 to send message, which is feed through Arduino Code, so there is no external wiring required Just connect with computer using USB cable and make sure about Wi-Fi credentials. If you are going further and use sensor and to send sensor data you can make wiring as sensor requirement. (Don’t forget to recode ESP32 for sensor data)
Components Required
- ESP32 Development Board (here we used DOIT Devkit V1)
- USB Cable
- Wi-Fi Network
Precheck
- Arduino IDE with ESP32 board support (If you are new to use ESP32 with Arduino IDE then Read Here, to get started)
- Install URLEncode Library in Arduino IDE, WiFi.h and HTTPClient.h comes built in with ESP32 board support.
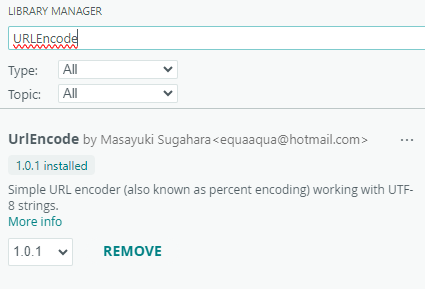
WhatsApp API
WhatsApp (meta) does not provide a direct public API for sending message from Microcontrollers. Instead here we will use third party service like CallMeBot.
Getting CallMeBot WhatsApp API
Go to callmebot.com website and and then send message under free WhatsApp API menu. there is a detailed setup instruction. Follow each step to get your API.
- CallMeBot website gives you a phone number like +34 684 73 40 44 you need to add this phone number to your phone contacts (Name it as you wish). Check the website because the number is dynamic and changes often.
- Send this message to the saved number “I allow callmebot to send me messages” using whatsApp.
- Wait until you get the API Messgage like, “API Activated for your phone number. Your APIKEY is 123123” from the bot. This API is for personal use only.
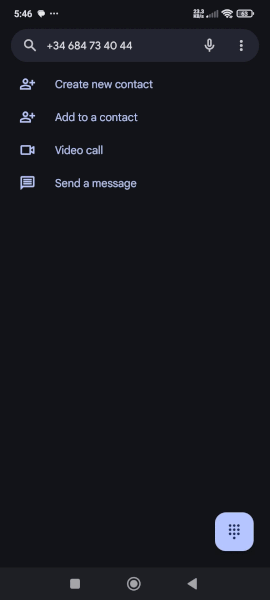
ESP32 WhatsApp Message Arduino Code
/*code from theoryCIRCUIT */ #include <WiFi.h> #include <HTTPClient.h> #include <URLEncode.h> // Include the library const char* ssid = "YOUR_WIFI_SSID"; const char* password = "YOUR_WIFI_PASSWORD"; const char* phoneNumber = "YOUR_PHONE_NUMBER"; const char* apiKey = "YOUR_API_KEY"; String message = "Hello from ESP32 Microcontroller "; // Dynamic or complex message URLEncode urlEncode; // Create an instance of the URLEncode class void setup() { Serial.begin(115200); WiFi.begin(ssid, password); Serial.print("Connecting to WiFi..."); while (WiFi.status() != WL_CONNECTED) { delay(500); Serial.print("."); } Serial.println("Connected to WiFi"); sendWhatsAppMessage(); } void loop() {} void sendWhatsAppMessage() { if (WiFi.status() == WL_CONNECTED) { HTTPClient http; // Encode the message String encodedMessage = urlEncode.encode(message.c_str()); // Construct the API URL String url = "https://api.callmebot.com/whatsapp.php?phone=" + String(phoneNumber) + "&text=" + encodedMessage + "&apikey=" + String(apiKey); http.begin(url); int httpCode = http.GET(); if (httpCode > 0) { String payload = http.getString(); Serial.println("HTTP Response code: " + String(httpCode)); Serial.println("Response: " + payload); } else { Serial.println("HTTP Request failed: " + String(http.errorToString(httpCode))); } http.end(); } else { Serial.println("WiFi not connected"); } }
Testing the Setup
Open the Arduino IDE and then past the above code and replace YOUR_WIFI_SSID, YOUR_WIFI_PASSWORD, YOUR_PHONE_NUMBER, YOUR_API_KEY, and message with your details. then upload the code.
Check the serial monitor at 115200 baud rate for verification either message successfully send or not.
Check the WhatsApp and you should receive the message on the specified phone number in international format.